React Best Practices: Component Architecture
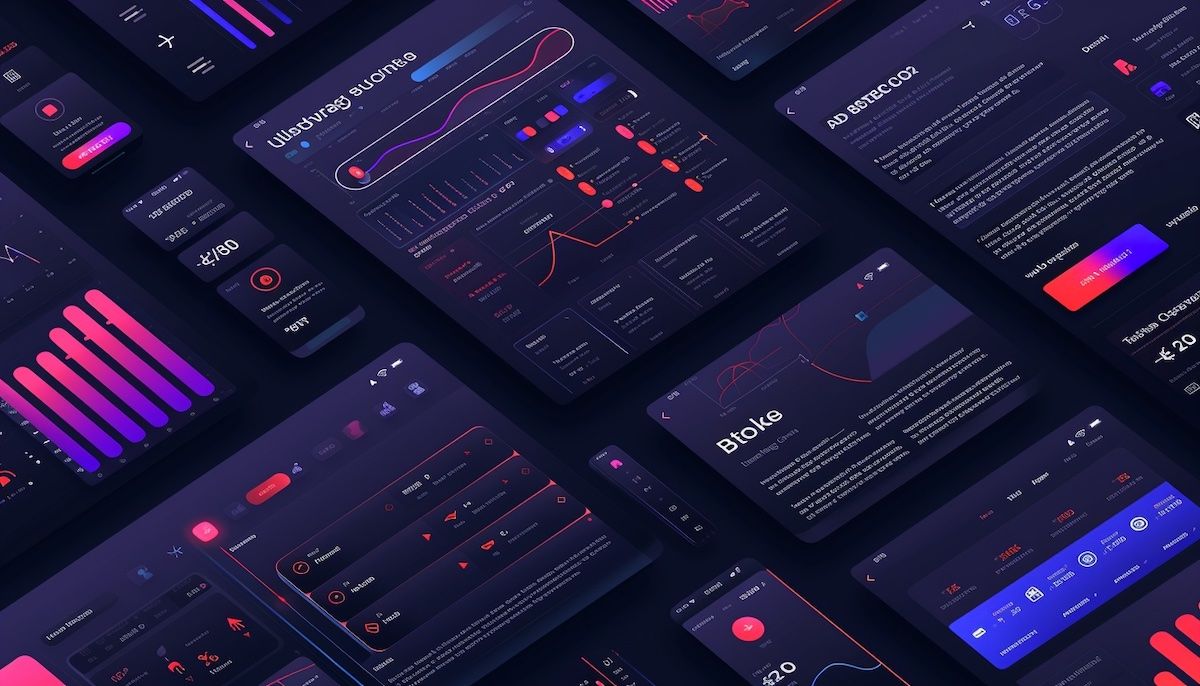
Building applications that are both scalable and maintainable is paramount.
Today, I want to dive deep into best practices for structuring components in a Next.js application using TypeScript. These practices are designed to enhance your project's robustness and ensure a seamless collaboration experience.
The concepts are relevant for any component based UI framework.
The Importance of Component Structure
In a typical Next.js project, where components play a central role, having a well-thought-out component structure is crucial. It not only aids in maintaining cleanliness in your codebase but also ensures that components are reusable and easy to test. Our focus here will be on distinguishing between "Pure Components" and "Connected Components" and illustrating how this separation can drastically improve your project's organization and efficiency.
Project Structure Overview
Before we delve into specifics, let's outline the basic directory structure we use:
src/app
: This directory contains all page-level components. Each component corresponds to a specific route in the application.src/components
: This is where all reusable UI components reside. "Connected" components act as data and state managers for the pure components.
Pure Components: The Building Blocks
Pure components are essentially the "dumb" components of your application. They focus solely on the UI, without concerning themselves with where the data comes from. Their main characteristics include:
- Data through props: Pure components receive all their data via props, maintaining their predictability and reusability.
- Statelessness: These components do not manage their state or side effects, which simplifies their testing and maintenance.
- Clarity in props definition: By using TypeScript, we define interfaces for props, which clarifies the expected data and enhances the development experience through type checking.
Example: A User Profile Component
// src/components/UserProfile.tsx
import React from 'react';
import Avatar from './Avatar';
import UserInfo from './UserInfo';
import UserActions from './UserActions';
export interface UserProfileProps {
imageUrl: string;
name: string;
bio: string;
onMessageClick: () => void;
onFollowClick: () => void;
}
export const UserProfile: React.FC<UserProfileProps> = ({
imageUrl,
name,
bio,
onMessageClick,
onFollowClick
}) => (
<div style={{ display: 'flex', flexDirection: 'column', alignItems: 'center', border: '1px solid #ccc', padding: '20px', borderRadius: '8px' }}>
<Avatar imageUrl={imageUrl} altText={name} />
<UserInfo name={name} bio={bio} />
<UserActions onMessageClick={onMessageClick} onFollowClick={onFollowClick} />
</div>
);
Connected Components: The Orchestrators
While pure components focus on presentation, connected components handle logic and state. These components are where the data fetching, state management, and data transformation take place, acting as the bridge between your application's data layer and its presentation layer.
Key Responsibilities:
- Manage data fetching: Connected components are responsible for retrieving data from external sources like APIs.
- State management: These components handle state complexities, particularly those affecting multiple child components.
- Data propagation: After processing, data is passed down to pure components through props.
Example: A "Connected" Component with State Management
To demonstrate a connected component in a Next.js application using TypeScript, we'll create a ConnectedUserProfile
component. This component will be responsible for fetching and managing user data, then passing this data to the UserProfile
component. We'll also handle some simple data transformation within the connected component to match the expected props of the UserProfile
.
Step 1: Mocking Data Fetching
First, let's assume we have a function to fetch user data from an API. In a real-world application, this would involve calling an API endpoint. Here, we'll simulate this with a timeout to mimic asynchronous data fetching.
Step 2: Transforming Data
We'll simulate a scenario where the API returns a user object with slightly different property names or structures than those expected by our UserProfile
pure component. We'll then transform this data to fit the pure component's prop requirements.
Step 3: ConnectedUserProfile Component
Here's how the ConnectedUserProfile
component could look:
// src/components/ConnectedUserProfile.tsx
import React, { useEffect, useState } from 'react';
import {UserProfile, UserProfileProps} from './UserProfile';
// Simulated user data fetching function
const fetchUserData = (): Promise<{ profile_picture_url: string; full_name: string; biography: string }> => {
return new Promise((resolve) => {
setTimeout(() => {
resolve({
profile_picture_url: 'https://example.com/avatar.jpg',
full_name: 'John Doe',
biography: 'Software Developer with a passion for front-end technologies.'
});
}, 1000);
});
};
const ConnectedUserProfile: React.FC = () => {
const [userData, setUserData] = useState<{ imageUrl: string; name: string; bio: string }>();
useEffect(() => {
fetchUserData().then(data => {
// Transforming data to fit UserProfile props
setUserData({
imageUrl: data.profile_picture_url,
name: data.full_name,
bio: data.biography
});
});
}, []);
// Handlers for user actions
const handleMessageClick = () => alert('Message User!');
const handleFollowClick = () => alert('Follow User!');
return (
<div>
{userData ? (
<UserProfile
imageUrl={userData.imageUrl}
name={userData.name}
bio={userData.bio}
onMessageClick={handleMessageClick}
onFollowClick={handleFollowClick}
/>
) : (
<p>Loading user profile...</p>
)}
</div>
);
};
export default ConnectedUserProfile;
Explanation
Data Fetching: fetchUserData
is a simulated asynchronous function that fetches user data. In a real application, this would likely involve API calls with fetch
or axios
.
State Management: userData
is managed locally within the component using useState
. It's initially undefined to handle the loading state until data is fetched and processed.
Data Transformation: The raw data from fetchUserData
is transformed to match the props expected by UserProfile
. This includes renaming fields and potentially formatting data.
Event Handlers: handleMessageClick
and handleFollowClick
are stubbed with simple alert functions. In practice, these would handle more complex logic such as navigating to a messaging screen or toggling a follow state.
Conditional Rendering: The component conditionally renders the UserProfile
once the data is available, otherwise displaying a loading message.
This example demonstrates the responsibilities of a connected component in separating data handling from presentation, which enhances code modularity and testability.
Cultivating Best Practices
To ensure that these practices are adopted and maintained:
- Perform regular code reviews: This helps reinforce standards and provides learning opportunities for developers.
- Keep documentation updated: Well-documented code and guidelines ensure that everyone on the team understands how to use and contribute to components correctly.
- Provide examples and templates: Templates act as a starting point for common patterns and reduce overhead in component creation.
By adopting these component structuring practices in your Next.js projects, you ensure that your applications are not only robust and efficient but also a pleasure to work on. Whether you're a seasoned developer or a newcomer to the team, these guidelines will help you contribute effectively and keep your project's quality consistently high.