Human Skills for Programming: Pattern Recognition
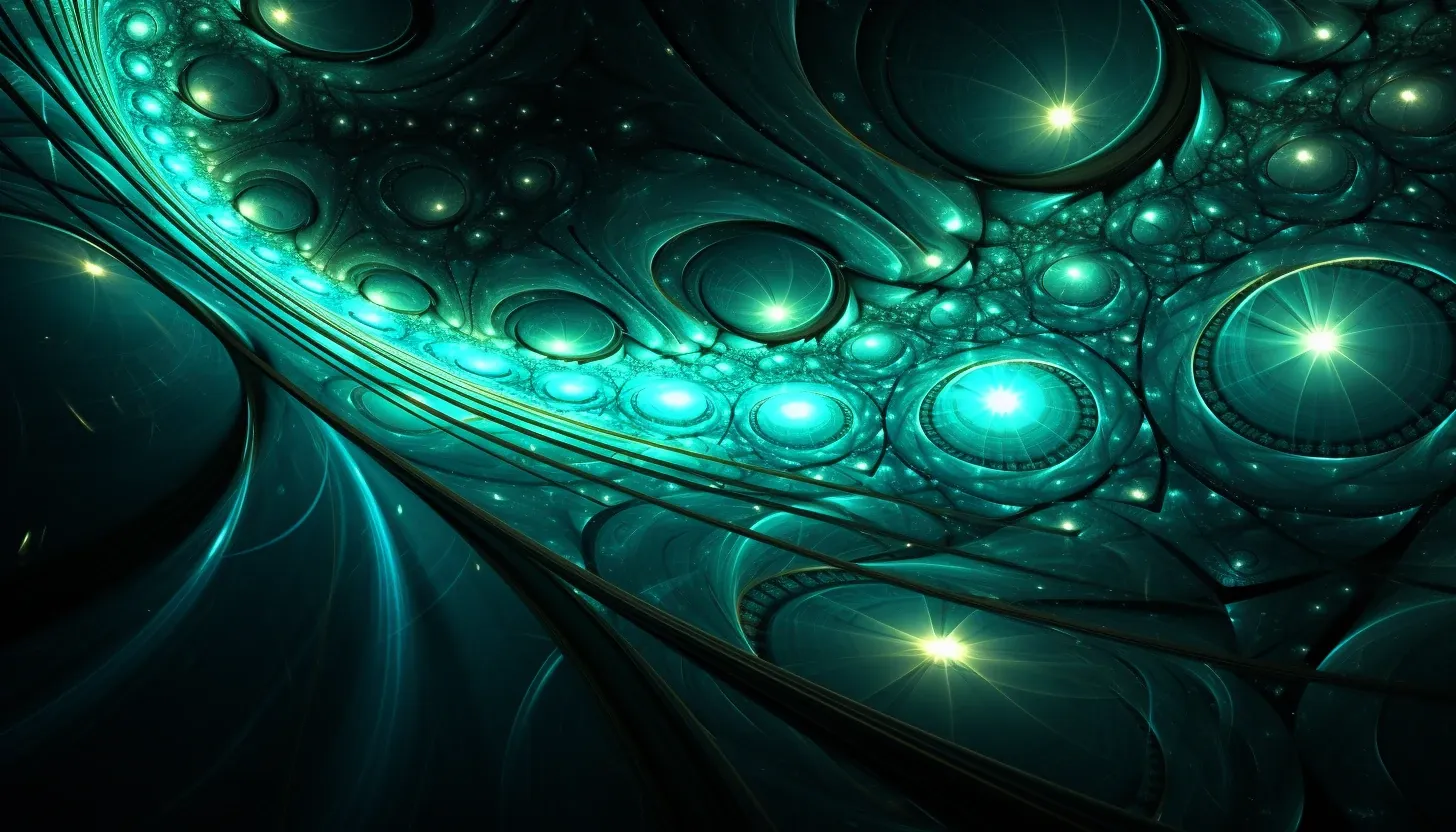
As I work with and mentor engineers and architects, there's one essential skill I see in the folks that tend to produce the greatest work.
They recognize patterns that can allow refactoring to simplify and reduce the amount of code required to produce quality results.
It's not critical that we create generalized functions for everything, but being able to recognize and create patterns will improve your overall abilities.
Here are some ways you can improve your pattern recognition skills to enhance your coding skills:
Identify Reusable Code Patterns
Look for recurring patterns in your code or in existing libraries and frameworks. Recognizing these patterns will help you write more concise and efficient code. For instance, you might find common design patterns like Singleton, Observer, Factory, etc. Once you identify these patterns, you can apply them in appropriate situations.
Learn from Others' Code
Study well-written and open-source code. Observe how experienced developers structure their code, handle edge cases, and apply design patterns. Identifying patterns in others' code will help you incorporate those practices into your own projects.
Spot Bug Patterns
Analyze your own code for recurring bugs or errors. If you notice common patterns in your mistakes, you can develop a checklist to avoid those issues in the future. This will enhance the reliability and stability of your code.
Think in Abstractions
Identify abstract concepts that apply across different programming languages and paradigms. Understanding abstractions will help you write more flexible, scalable, and maintainable code.
Practice Pattern Recognition Puzzles
Engage in coding challenges and puzzles that encourage pattern recognition skills. Platforms like LeetCode, HackerRank, and Codeforces offer a variety of coding problems that require pattern identification to solve efficiently.
Apply Design Patterns
Learn and apply common design patterns in your coding projects. Design patterns provide proven solutions to recurring software design problems. Familiarity with design patterns will make your code more structured and easier to maintain.
Stay Curious and Experiment
Keep an open mind and continuously explore new programming concepts, languages, and paradigms. Experimenting with different techniques will expose you to various patterns and improve your coding versatility.
Collaborate and Review Code
Engage with other developers, participate in code reviews, and discuss coding approaches. Exposure to different coding styles and practices will enhance your pattern recognition skills.
Furthering Your Skills
Master Algorithmic Patterns
Recognize common algorithms and data structures, such as sorting algorithms, tree traversal, dynamic programming, and graph algorithms. Understanding these patterns will enable you to choose the most appropriate algorithm for specific programming tasks, leading to more efficient and optimized solutions.
Analyze Performance Patterns
Recognize performance bottlenecks and inefficiencies in your code. This could involve identifying loops that run too many times, redundant computations, or memory leaks. Being able to identify these patterns will aid in improving the overall performance of your applications.
Remember that pattern recognition skills improve with experience and practice. The more you code and analyze different patterns, the better equipped you'll be to write clean, efficient, and robust code.